Quick start
This guide will walk you through the basic steps of generating AI fashion models for fashion ecommerce with AI Fashion Model API.
Let's say you have an image of a female model and want to change the model while keeping the same pose and outfit as shown in the image below.
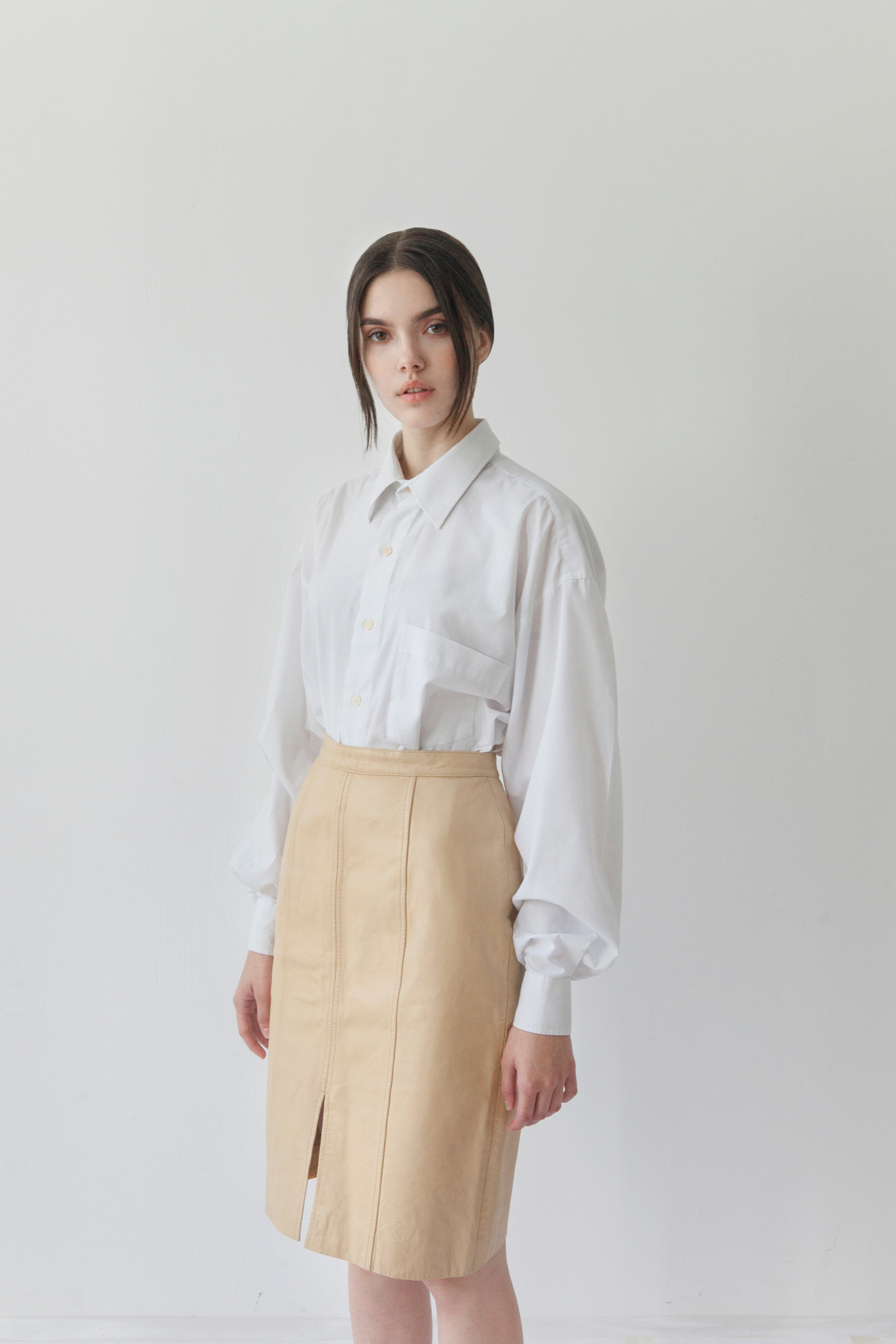
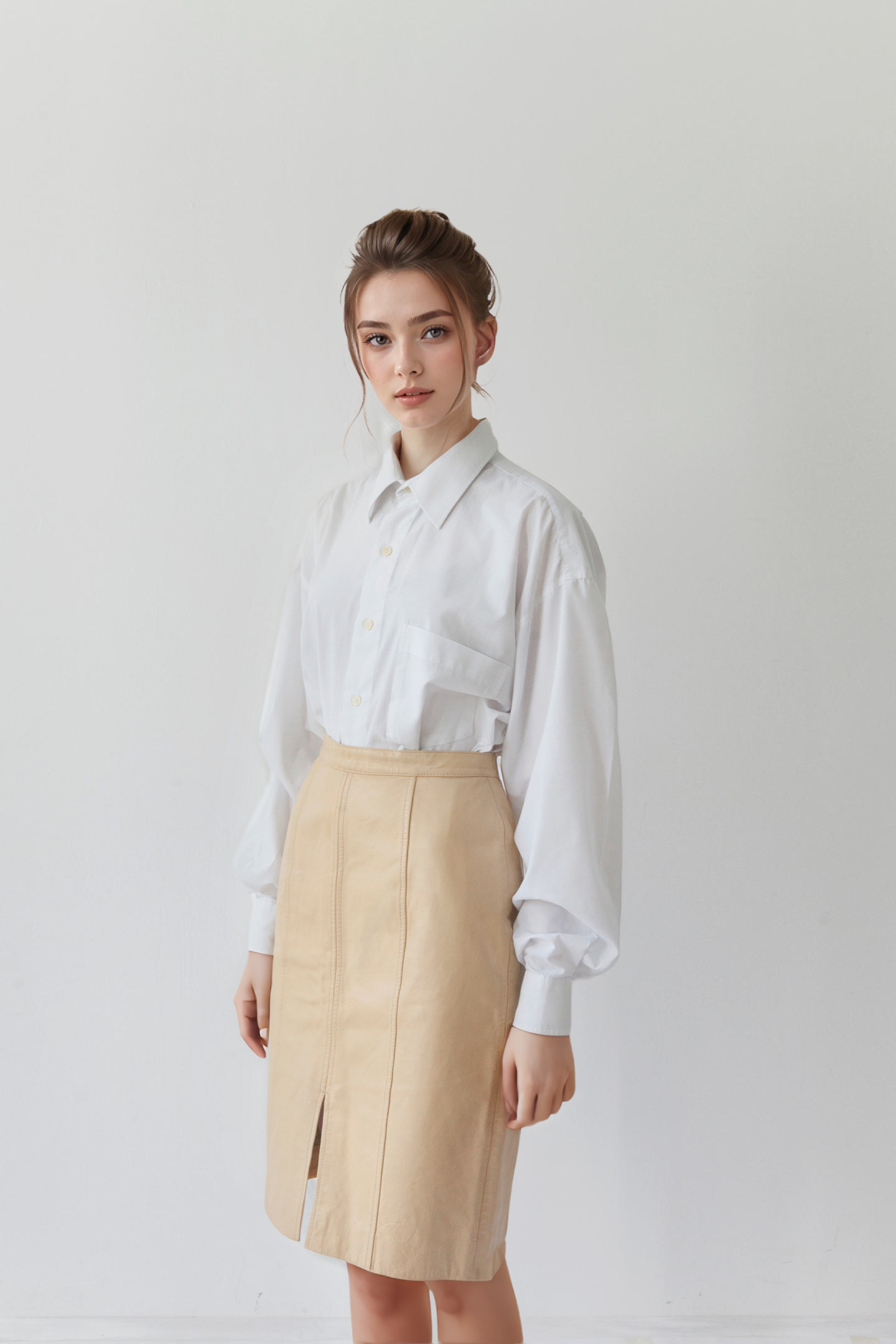
Complete in one move
Before completing the step-by-step tutorial, you can quickly experience the API through the following component, and you can also copy the
source code implemented in different languages and run it directly. Remember to replace YOUR_API_KEY
in the source code with your own API Key.
package main
import (
"bytes"
"encoding/json"
"fmt"
"io"
"log"
"net/http"
"time"
)
func main() {
taskId, err := createTask()
if err != nil {
log.Fatalln("failed to create task:", err.Error())
return
}
log.Println("task created successfully:", taskId)
var results []TaskResult
for {
taskInfo, err := checkTask(taskId)
if err != nil {
log.Fatalln("failed to check task:", err.Error())
return
}
if taskInfo.Status == "error" {
log.Fatalln("task failed:", taskInfo.Message)
return
}
log.Printf("task status: %s, progress: %d%%\n", taskInfo.Status, taskInfo.Progress)
if taskInfo.Status == "pending" || taskInfo.Status == "running" {
time.Sleep(3 * time.Second)
continue
}
if taskInfo.Status == "success" {
results = taskInfo.Results
break
}
}
log.Println("task completed successfully")
for _, result := range results {
log.Println("Result image: ", result.DownloadUrl)
}
}
const apiKey = "YOUR_API_KEY"
type ResponseBody[D any] struct {
Code int `json:"code"`
Message string `json:"message"`
Data D `json:"data,omitempty"`
}
// createTask creates an asynchronous task to generate AI fashion models
func createTask() (taskId string, err error) {
data := map[string]any{
"image": "https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png",
"modelId": "002",
"sceneType": "preset",
"sceneId": "035",
}
jsonData, err := json.Marshal(data)
if err != nil {
return "", err
}
request, err := http.NewRequest("POST", "https://open.vmake.ai/api/v4/image/ai-fashion-model/clothing-tasks", bytes.NewBuffer(jsonData))
if err != nil {
return "", err
}
request.Header.Set("X-Api-Key", apiKey)
request.Header.Set("Content-Type", "application/json")
client := &http.Client{}
response, err := client.Do(request)
if err != nil {
return "", err
}
defer func() { err = response.Body.Close() }()
if response.StatusCode != http.StatusOK {
return "", fmt.Errorf("failed to create task: %s", response.Status)
}
bodyBytes, err := io.ReadAll(response.Body)
if err != nil {
return "", err
}
responseBody := new(ResponseBody[map[string]any])
err = json.Unmarshal(bodyBytes, responseBody)
if err != nil {
return "", err
}
code := responseBody.Code
message := responseBody.Message
if code != 0 {
return "", fmt.Errorf("failed to create task: %s", message)
}
taskId = responseBody.Data["taskId"].(string)
return taskId, err
}
type TaskInfo struct {
TaskId string `json:"taskId"`
Status string `json:"status"`
Message string `json:"message"`
Progress int `json:"progress"`
Results []TaskResult `json:"results,omitempty"`
}
type TaskResult struct {
Width int `json:"width"`
Height int `json:"height"`
FileSize int `json:"fileSize"`
DownloadUrl string `json:"downloadUrl"`
}
func checkTask(taskId string) (taskInfo *TaskInfo, err error) {
request, err := http.NewRequest("GET", fmt.Sprintf("https://open.vmake.ai/api/v4/image/ai-fashion-model/clothing-tasks/%s", taskId), nil)
if err != nil {
return nil, err
}
request.Header.Set("X-Api-Key", apiKey)
client := &http.Client{}
response, err := client.Do(request)
if err != nil {
return nil, err
}
defer func() { err = response.Body.Close() }()
if response.StatusCode != http.StatusOK {
return nil, fmt.Errorf("failed to check task: %s", response.Status)
}
bodyBytes, err := io.ReadAll(response.Body)
if err != nil {
return nil, err
}
responseBody := new(ResponseBody[TaskInfo])
err = json.Unmarshal(bodyBytes, responseBody)
if err != nil {
return nil, err
}
code := responseBody.Code
message := responseBody.Message
if code != 0 {
return nil, fmt.Errorf("failed to create task: %s", message)
}
return &responseBody.Data, err
}
Step by step
To deep dive into the process of generating AI fashion models, you can follow the step-by-step tutorial below.
Prepare input image
The AI Fashion Model API requires one image as input:
- The original image of clothing model that you want to change.
In this tutorial, the API automatically generate the mask image. You only need to provide the original image. Here is the original image URL that you will use in this tutorial: https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png (opens in a new tab)
The request body should look like below:
{
"image": "https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png",
}
Choose a fashion model
The API provides a list of fashion models that you can choose from. Below are some preset models, you can also preview all available models in the Preset Models page.
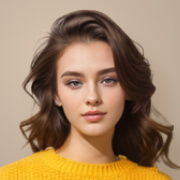
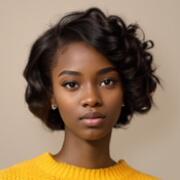
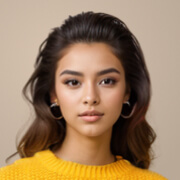
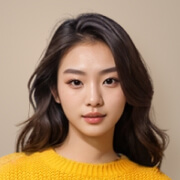
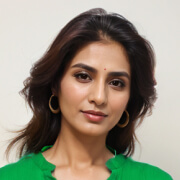
Suppose you want to use the model "Clara" in this tutorial. The request body should look like below:
{
"image": "https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png",
"modelId": "002"
}
Choose scene type
The API support four types of background:
- original: Indicates that the generated image will use the original background from the input image.
- preset: Indicates that the generated image will use a scene based on the selected
sceneId
. - color: Indicates that the generated image will use a solid color background corresponding to the specified color value.
- none: Indicates that the generated image will have a transparent background.
View all available scenes in the Preset Scenes page.
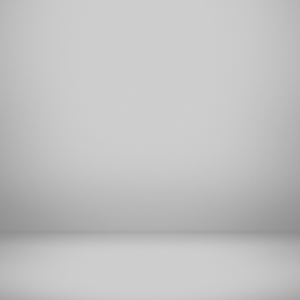
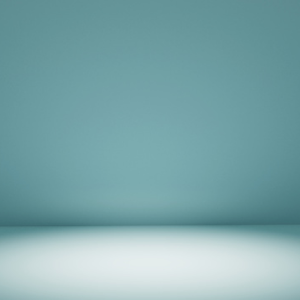
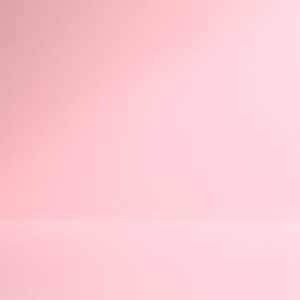
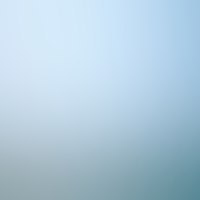
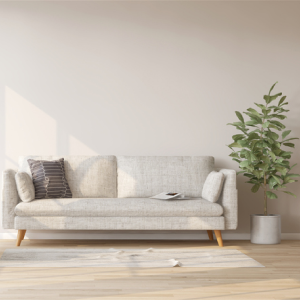
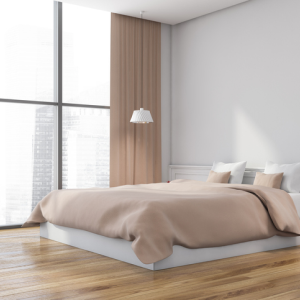
Suppose you want to generate the model with preset scene type. And the preset scene ID is "035". The request body should look like below:
{
"image": "https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png",
"modelId": "002",
"sceneType": "preset",
"sceneId": "035"
}
Create asynchronous task
So far, the request body is ready as shown below:
{
"image": "https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png",
"modelId": "002",
"sceneType": "preset",
"sceneId": "035"
}
Now, you can send a POST request to the API endpoint to create an asynchronous task. Since generating AI fashion models is a time-consuming process, the API will return a task ID that you can use to check the status of the task and download the generated model images.
After sending the request, the API will return a response body with the task ID that you can use to check the status of the task and download the generated model images. The response body should look like below:
{
"code": 0,
"message": "Success",
"data": {
"taskId": "0f1c2d3e-4f5g-6h7i-8j9k-a1b2c3d4e5f6"
}
}
Let's see how to send the request in different programming languages.
curl -X POST \
-H "X-Api-Key: YOUR_API_KEY" \
-H "Content-Type: application/json" \
-d '{
"image": "https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png",
"modelId": "002",
"sceneType": "preset",
"sceneId": "035"
}' \
https://open.vmake.ai/api/v4/image/ai-fashion-model/clothing-tasks
Check task status
So far, you have successfully created an asynchronous task and received a task ID. Now, you can send a GET request to the API endpoint to check the status of the task in fixed intervals until the task is completed.
After sending the request, the API will return a response body with the task status in the data
field like below:
{
"code": 0,
"message": "Success",
"data": {
"taskId": "0f1c2d3e-4f5g-6h7i-8j9k-a1b2c3d4e5f6",
"status": "pending",
"progress": 0,
"results": null
}
}
- taskId: The asynchronous task ID.
- status: The status indicates the current status of the task.
- progress: The progress indicates the percentage of the task completion.
- results: The results present only when the status is
success
.
For more information about the task status, you can refer to the Task object documentation. Now let's see how to send the request in different programming languages.
curl -X GET \
-H "X-Api-Key: YOUR_API_KEY" \
https://open.vmake.ai/api/v4/image/ai-fashion-model/clothing-tasks/0f1c2d3e-4f5g-6h7i-8j9k-a1b2c3d4e5f6
Ready to run
Now, you have known how to create an asynchronous task and check the task status. Let's put all the code together and run it.
package main
import (
"bytes"
"encoding/json"
"fmt"
"io"
"log"
"net/http"
"time"
)
func main() {
taskId, err := createTask()
if err != nil {
log.Fatalln("failed to create task:", err.Error())
return
}
log.Println("task created successfully:", taskId)
var results []TaskResult
for {
taskInfo, err := checkTask(taskId)
if err != nil {
log.Fatalln("failed to check task:", err.Error())
return
}
if taskInfo.Status == "error" {
log.Fatalln("task failed:", taskInfo.Message)
return
}
log.Printf("task status: %s, progress: %d%%\n", taskInfo.Status, taskInfo.Progress)
if taskInfo.Status == "pending" || taskInfo.Status == "running" {
time.Sleep(3 * time.Second)
continue
}
if taskInfo.Status == "success" {
results = taskInfo.Results
break
}
}
log.Println("task completed successfully")
for _, result := range results {
log.Println("Result image: ", result.DownloadUrl)
}
}
const apiKey = "YOUR_API_KEY"
type ResponseBody[D any] struct {
Code int `json:"code"`
Message string `json:"message"`
Data D `json:"data,omitempty"`
}
// createTask creates an asynchronous task to generate AI fashion models
func createTask() (taskId string, err error) {
data := map[string]any{
"image": "https://x-design-release.stariidata.com/poster/d8f92d37a0569bd6c3a7f27e2882fd74.png",
"modelId": "002",
"sceneType": "preset",
"sceneId": "035",
}
jsonData, err := json.Marshal(data)
if err != nil {
return "", err
}
request, err := http.NewRequest("POST", "https://open.vmake.ai/api/v4/image/ai-fashion-model/clothing-tasks", bytes.NewBuffer(jsonData))
if err != nil {
return "", err
}
request.Header.Set("X-Api-Key", apiKey)
request.Header.Set("Content-Type", "application/json")
client := &http.Client{}
response, err := client.Do(request)
if err != nil {
return "", err
}
defer func() { err = response.Body.Close() }()
if response.StatusCode != http.StatusOK {
return "", fmt.Errorf("failed to create task: %s", response.Status)
}
bodyBytes, err := io.ReadAll(response.Body)
if err != nil {
return "", err
}
responseBody := new(ResponseBody[map[string]any])
err = json.Unmarshal(bodyBytes, responseBody)
if err != nil {
return "", err
}
code := responseBody.Code
message := responseBody.Message
if code != 0 {
return "", fmt.Errorf("failed to create task: %s", message)
}
taskId = responseBody.Data["taskId"].(string)
return taskId, err
}
type TaskInfo struct {
TaskId string `json:"taskId"`
Status string `json:"status"`
Message string `json:"message"`
Progress int `json:"progress"`
Results []TaskResult `json:"results,omitempty"`
}
type TaskResult struct {
Width int `json:"width"`
Height int `json:"height"`
FileSize int `json:"fileSize"`
DownloadUrl string `json:"downloadUrl"`
}
func checkTask(taskId string) (taskInfo *TaskInfo, err error) {
request, err := http.NewRequest("GET", fmt.Sprintf("https://open.vmake.ai/api/v4/image/ai-fashion-model/clothing-tasks/%s", taskId), nil)
if err != nil {
return nil, err
}
request.Header.Set("X-Api-Key", apiKey)
client := &http.Client{}
response, err := client.Do(request)
if err != nil {
return nil, err
}
defer func() { err = response.Body.Close() }()
if response.StatusCode != http.StatusOK {
return nil, fmt.Errorf("failed to check task: %s", response.Status)
}
bodyBytes, err := io.ReadAll(response.Body)
if err != nil {
return nil, err
}
responseBody := new(ResponseBody[TaskInfo])
err = json.Unmarshal(bodyBytes, responseBody)
if err != nil {
return nil, err
}
code := responseBody.Code
message := responseBody.Message
if code != 0 {
return nil, fmt.Errorf("failed to create task: %s", message)
}
return &responseBody.Data, err
}
In the code snippet above, you first create an asynchronous task to generate AI fashion models. Then, you check the task status in fixed intervals until the task is completed. Finally, you print the download URLs of the generated images.
Note: Since AI fashion model generation is a time-consuming process, you may need to wait for a while until the task is completed.
View the results
After the task completed successfully, you can view the generated images by using the download URLs in the results. Below shows the generated images with different scenes.
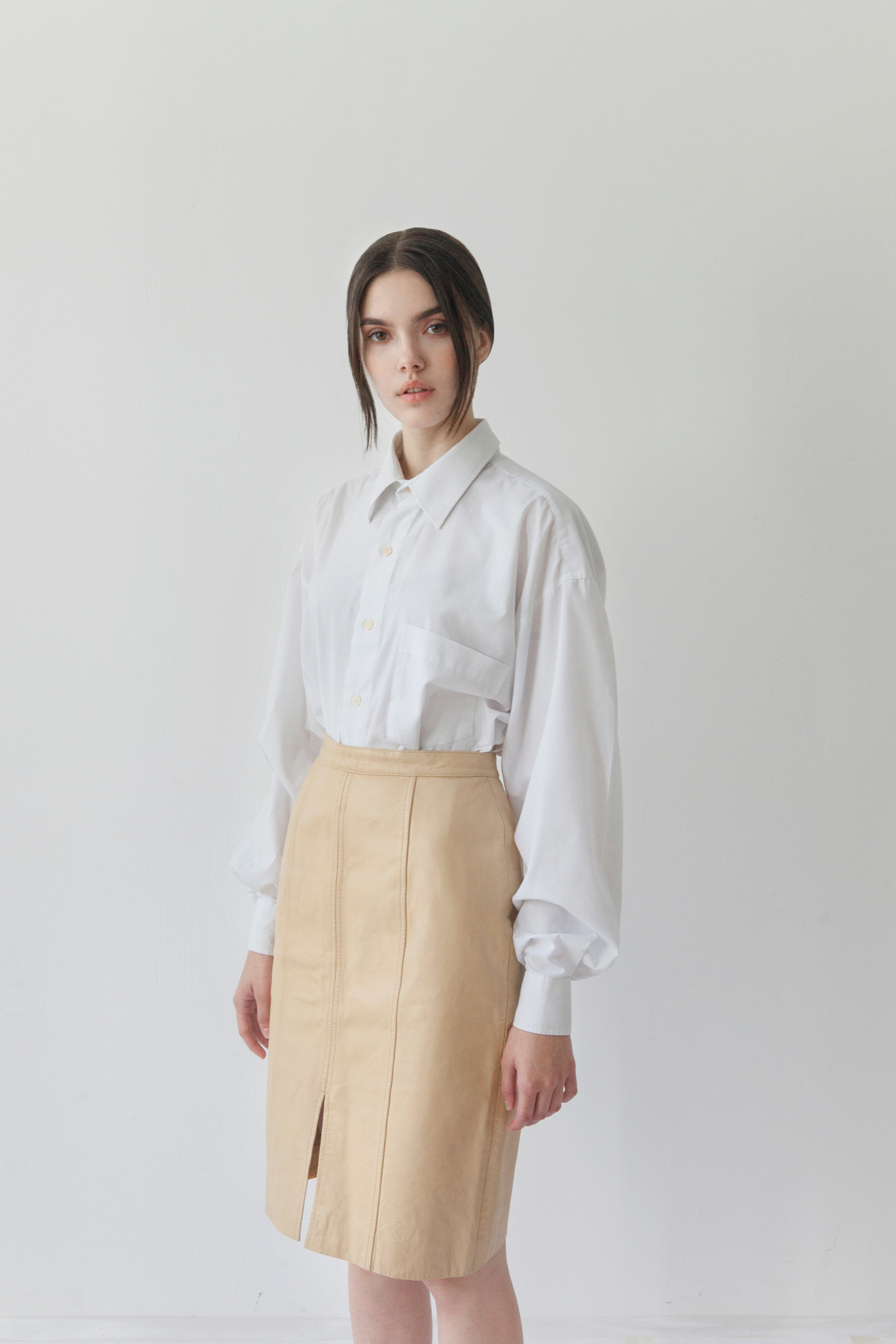
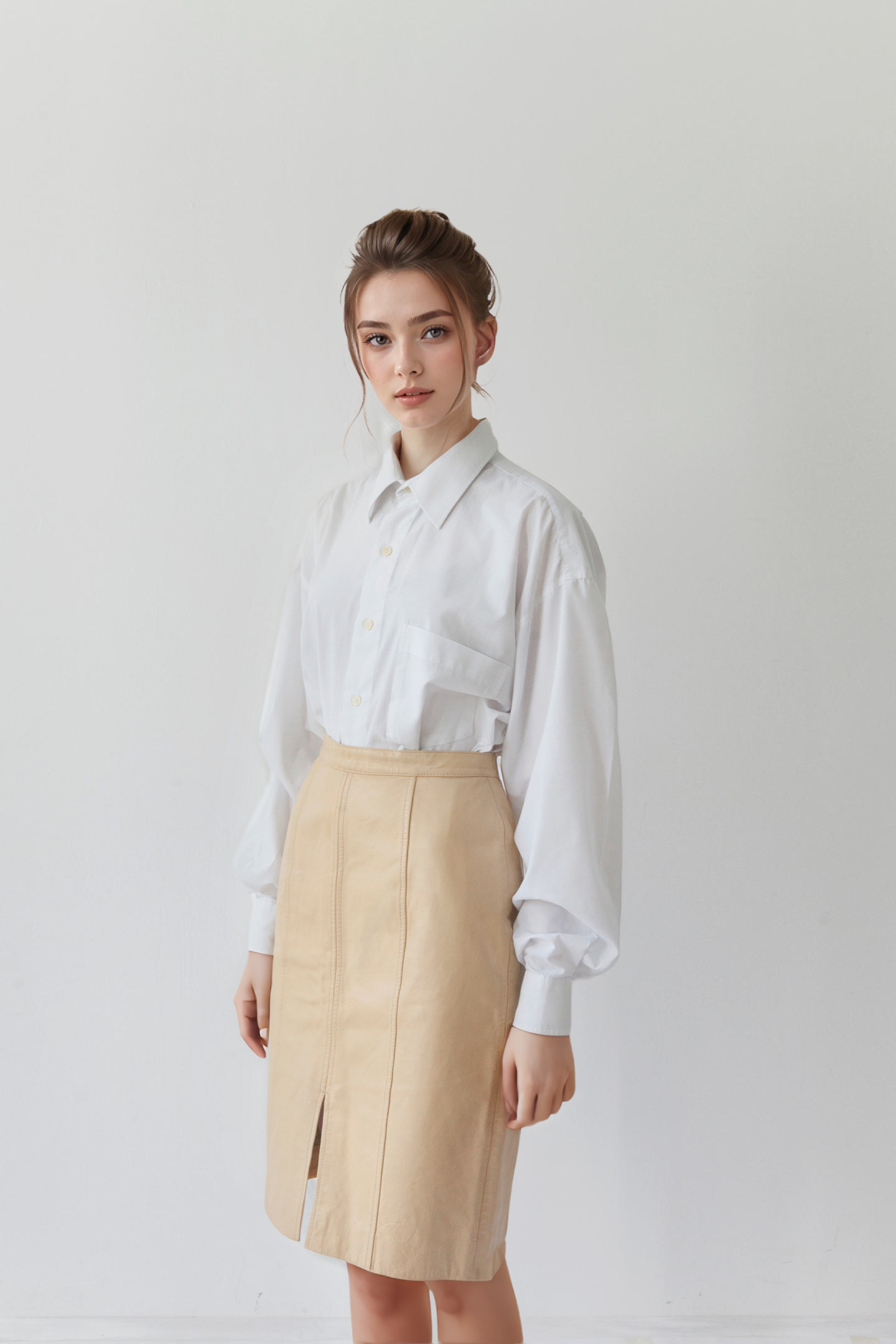
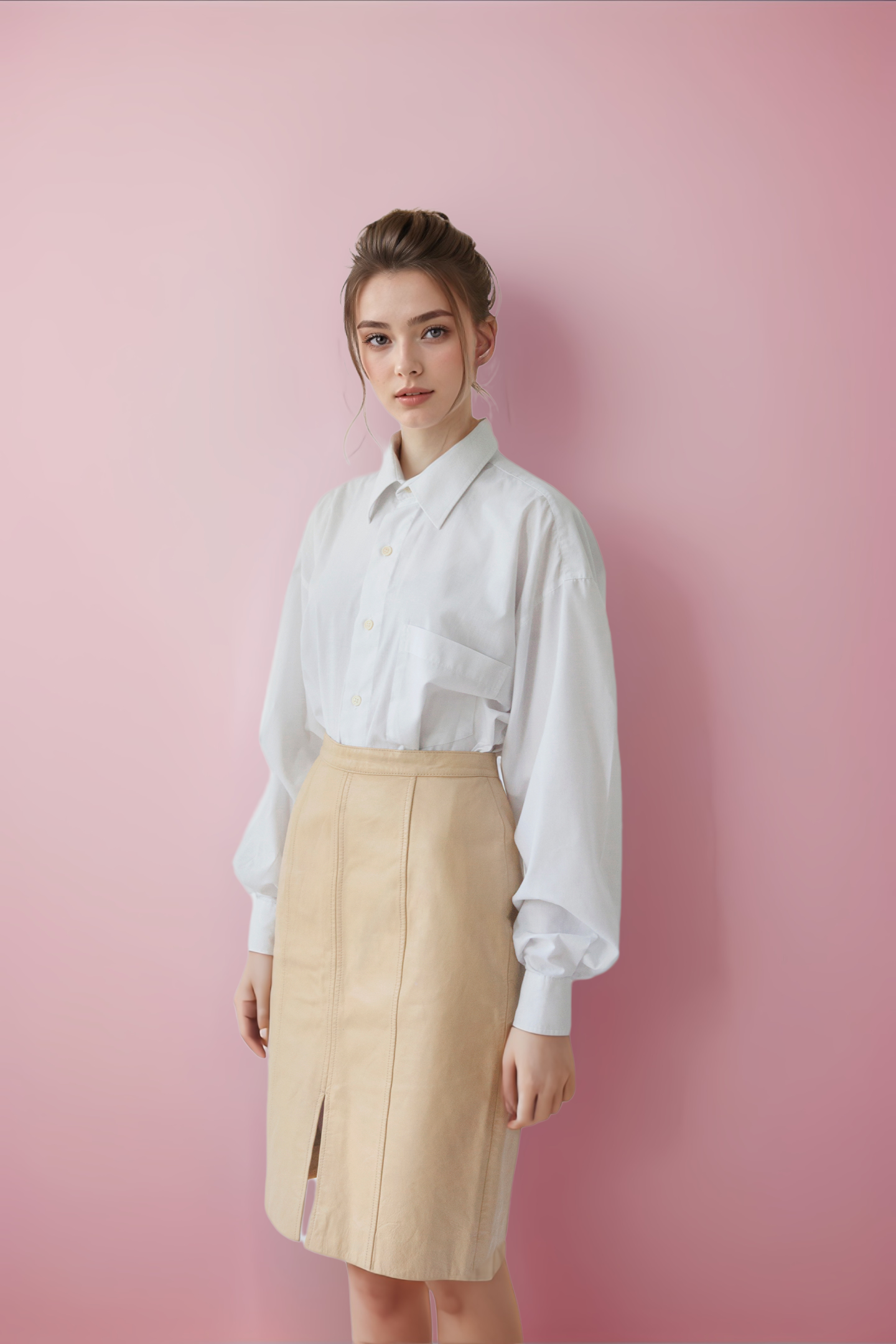
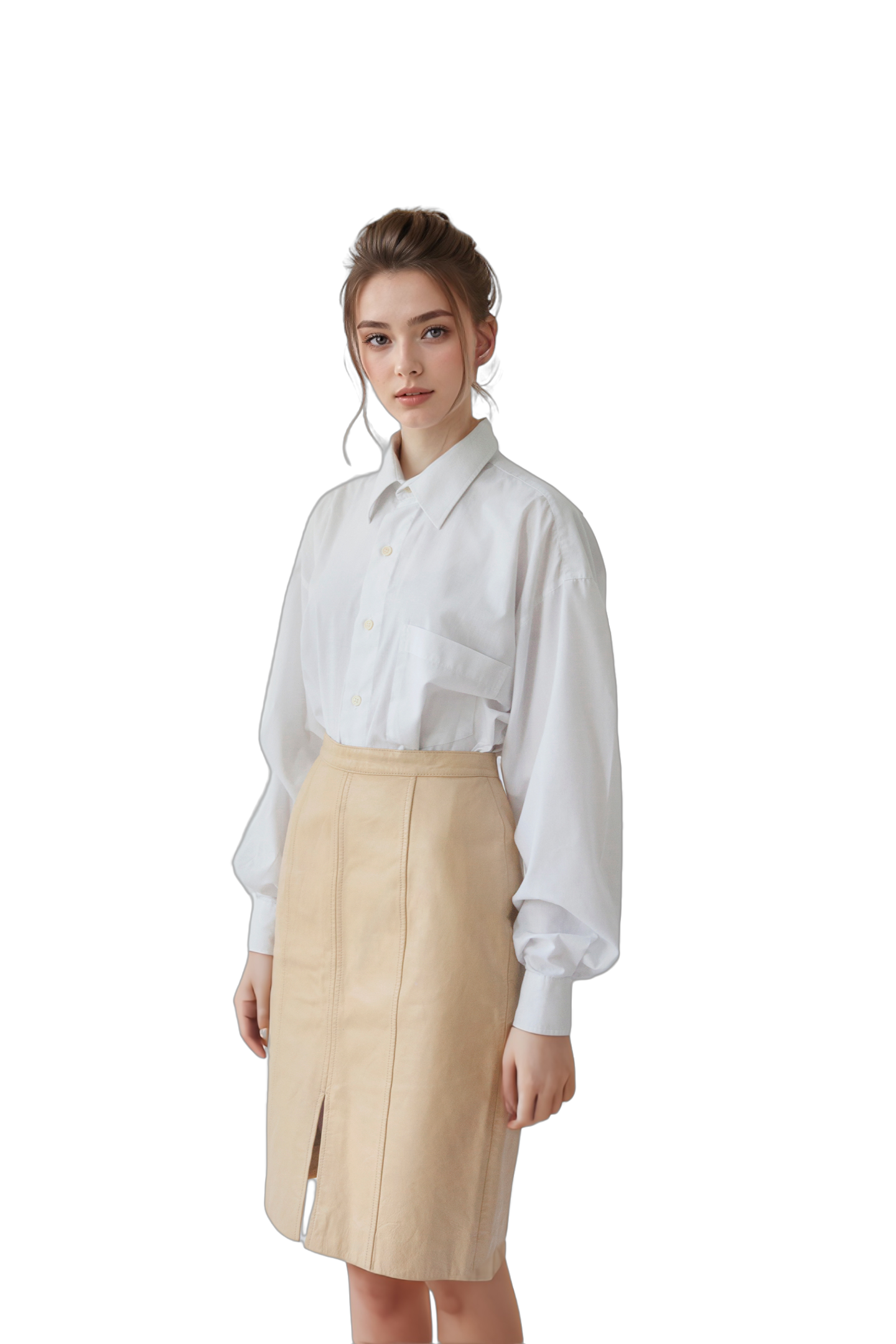
Congratulations! You have successfully generated AI fashion models with different scenes using the Vmake AI Fashion Model API.
Conclusion
In this tutorial, you have learned how to create an asynchronous task to generate AI fashion models and check the task status until the task is completed. You have also seen how to view the generated images with different scenes. In the next tutorial, you will learn how to generate AI fashion models with customized masks.